Making Pins and Callouts in Apple Maps Accessible
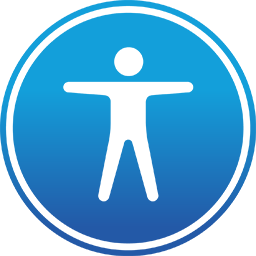
Pins (aka location markers) and Callouts on instances of MKMapView are not automatically accessible to blind users via iOS’s VoiceOver feature. The impact of this is that while blind users may be able to load your app’s map they won’t be able to find any of the content that you’ve placed on the map. Thankfully, there’s an easy fix for this using the stock Accessibility framework that Apple provides in iOS.
Update your MKMapViewDelegate’s callOut and set annotationView objects to be accessible.
- (MKAnnotationView *)mapView:(MKMapView *)mv viewForAnnotation:(id )annotation
{
NSString *identifier = @"PINIDENTIFIER";
MKPinAnnotationView *annotationView = (MKPinAnnotationView *)[mapView dequeueReusableAnnotationViewWithIdentifier:identifier];
if (annotationView == nil)
{
annotationView = [[MKPinAnnotationView alloc] initWithAnnotation:annotation reuseIdentifier:identifier];
annotationView.canShowCallout = true;
UIButton *callOut = [UIButton buttonWithType:UIButtonTypeDetailDisclosure];
[callOut setAccessibilityValue:@"Tap To Perform Some Action"];
annotationView.rightCalloutAccessoryView = callOut;
[annotationView setIsAccessibilityElement:YES];
annotationView.accessibilityValue = @"Tap this pin show a callout with more information.";
}
return annotationView;
}
Make sure any UIView that the MKMapView may be contained in the XIB has it’s accessibility option set to NO. Not doing so will cause the map view to be accessible, when it’s only the pins and callouts that are desired.